In this guide, I’ll show you how to master Linux shell scripting. It’s key for automating tasks and managing systems in Unix/Linux. We’ll dive into the Bash shell, scripting basics, variables, and more.
You’ll learn about loops, conditional statements, and how to schedule and debug scripts. By the end, you’ll be ready to write your own Linux shell scripts.
Key Takeaways
- Understand the fundamentals of Linux shell scripting using the Bash shell
- Learn to create and execute Bash scripts for automation and system management
- Explore variables, conditional statements, and loops to write more sophisticated scripts
- Discover advanced techniques for scheduling and debugging Bash scripts
- Gain practical experience through hands-on examples and real-world Linux administration scripts
Understanding the Bash Shell
The Bash shell (Bourne-Again SHell) is a key Unix/Linux shell. It’s the default in many Linux systems. A shell lets you interact with your system through a command-line interface. You can run commands, move around the file system, and automate tasks.
While Bash is a specific shell, others like Korn shell (ksh), C shell (csh), and Z shell (zsh) also exist. Each has its own way of working and features.
Overview of Bash Shell and Command Line Interface
The Bash shell is the go-to in most Linux systems and comes pre-installed. It’s a powerful tool for many tasks, from simple file management to complex system tasks. The command-line interface of the Bash shell lets you work directly with your system. You don’t need a graphical user interface (GUI).
Pre-requisites for Bash Scripting
- You need access to a running Linux system or a way to use the Linux command line. This could be through a virtual machine, a cloud environment like Replit, or the Windows Subsystem for Linux (WSL).
- Basic command line skills are necessary. You should know how to use commands like
ls
,cd
,mkdir
, andecho
. - Some programming knowledge is helpful. Knowing about variables, functions, and control structures will aid in understanding this tutorial.
“Understanding Bash scripting can provide a strong foundation for learning other scripting languages and tools such as Python and Ansible.”
Learning the Bash shell and its command-line interface is a great start. It will help you become skilled in Linux shell scripting and automating tasks on your system.
Introduction to Bash Scripting
Definition of Bash Scripting
Bash scripting is short for “Bourne Again Shell” scripting. It involves creating a file with a series of commands. These commands can be run by the Bash shell program. This tool automates actions like moving to a directory or launching a process.
It’s a key part of Linux, helping system administrators and developers. They use it to make tasks faster and less prone to errors.
Advantages of Bash Scripting
Bash scripting has many benefits in the Linux world:
- Automation: It automates tasks, saving time and reducing errors.
- Portability: Scripts work on various Unix/Linux platforms and Windows with emulators.
- Flexibility: Scripts can be customized and combined with other languages for more power.
- Accessibility: It’s easy to learn and doesn’t need special tools. Most systems have a Bash interpreter.
- Integration: Scripts work with tools like databases and web servers, enabling complex tasks.
- Debugging: Debugging is straightforward, thanks to Bash’s built-in tools.
Bash scripting is a must-have skill for Linux users. It makes tasks more efficient and manageable.
Getting Started with Bash Scripting
Learning the Bash shell and command line is key to mastering Linux shell scripting. The Bash shell, or “Bourne-Again SHell,” is very common in Linux. Knowing the basic Bash commands and their syntax is essential before you start scripting.
Running Bash Commands from the Command Line
The shell prompt, shown as [username@host ~]$
, is where you enter Bash commands. Some important commands to start with are:
date
: Shows the current date and timepwd
: Shows the current directoryls
: Lists the current directory’s contentsecho
: Prints text or variable values to the terminal
For more info on any Bash command, use man
. It gives detailed info on the command’s syntax and options.
Creating and Executing Bash Scripts
To make a Bash script, follow these steps:
- Choose a file name with a
.sh
extension, though it’s not required. - Add a shebang line: The first line should be
#!/bin/bash
, which tells Bash where to find the interpreter. - Write your script: Put your Bash commands, one per line.
- Make the script executable: Use
chmod
to add execution rights, likechmod u+x script.sh
. - Run the script: You can run it with
./script.sh
,bash script.sh
, orsh script.sh
.
By doing these steps, you can create and run your own Bash scripts. This helps automate tasks and makes your Linux workflow smoother.
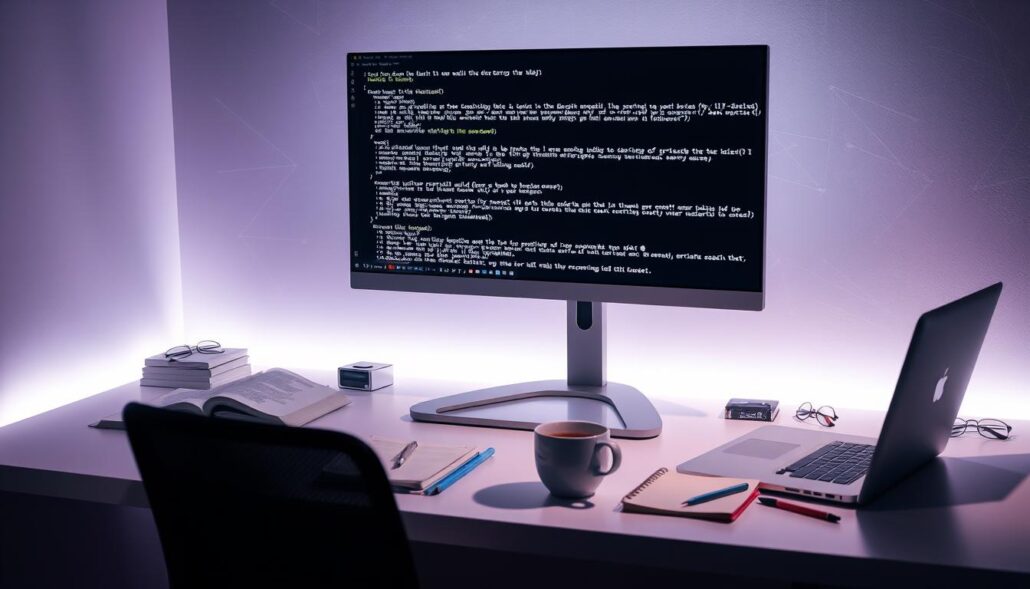
“Bash scripting is a powerful tool for automating tasks and improving efficiency in the Linux environment. It’s a skill that every Linux user should consider learning.”
Bash Scripting Basics
Comments in Bash Scripting
It’s key to document your Bash scripts for better understanding and teamwork. In Bash, comments start with `#` and are skipped by the interpreter. It’s smart to include comments to explain your script’s purpose and guide users.
Well-commented Bash scripts are simpler to grasp, upkeep, and share.
Variables and Data Types in Bash
Bash variables help store and work with data in your scripts. Bash doesn’t have strict data types like some languages. Variables can hold numbers, single characters, or strings.
To set a variable’s value, use `variable_name=value. To see a variable’s value, start it with `$`, like `echo $variable_name. Bash also has basic math with `$(()).
Bash Data Type | Description |
---|---|
Integers | Whole numbers, both positive and negative, such as 42 or -7. |
Floating-point | Decimal numbers, like 3.14 or -2.5. |
Strings | Sequences of characters, such as “Hello, world!” or “Bash scripting”. |
Arrays | Collections of values, similar to lists, indexed by numbers or strings. |
Correct variable assignment and variable access are vital for good Bash scripting. Knowing the Bash data types helps pick the right variables and perform needed actions.
Working with Input and Output
In Bash scripting, handling input and output is key. Bash scripts can take in Bash script input and capture Bash script output from commands. This makes it easy to create dynamic and flexible automation workflows.
The read
command lets you get user input in Bash scripts. It prompts the user for input and saves it in a variable. Using read
, you can get important info from the user and use it in your script.
To capture command output, Bash has a feature called command substitution. You put the command in backticks (`
) or use the $()
syntax. The command’s output is saved in a variable, making it easy to add to your script. This method is great for making Bash automation more dynamic and responsive.
Command | Description |
---|---|
read | Prompts the user for input and stores it in a variable |
`command` or $(command) | Captures the output of a command and stores it in a variable |
Learning to handle Bash script input and Bash script output is crucial. It lets you make Bash scripts that are interactive and flexible. They can respond to user needs and use the results of different commands. This skill is vital for creating effective and user-friendly Bash automation solutions.
Control Structures in Bash
As a Bash scripting fan, I’ve learned how control structures make scripts more dynamic. They let me decide what code to run based on conditions. The `if` statement is key, letting me choose between different paths based on true or false conditions.
Using `elif` and `else`, I can make more complex decisions. This lets me handle a variety of situations, from simple to complex.
Loops and Branching
Loops are also crucial for me. The `for` loop helps me work through lists easily. The `while` loop lets me keep doing something as long as a condition is met.
The `case` statement is a game-changer. It makes handling many conditions simple and organized. This has made my scripts cleaner and easier to maintain.
Learning about control structures has taken my Bash scripting to the next level. I can automate tasks, improve workflows, and create advanced scripts. The possibilities are endless.
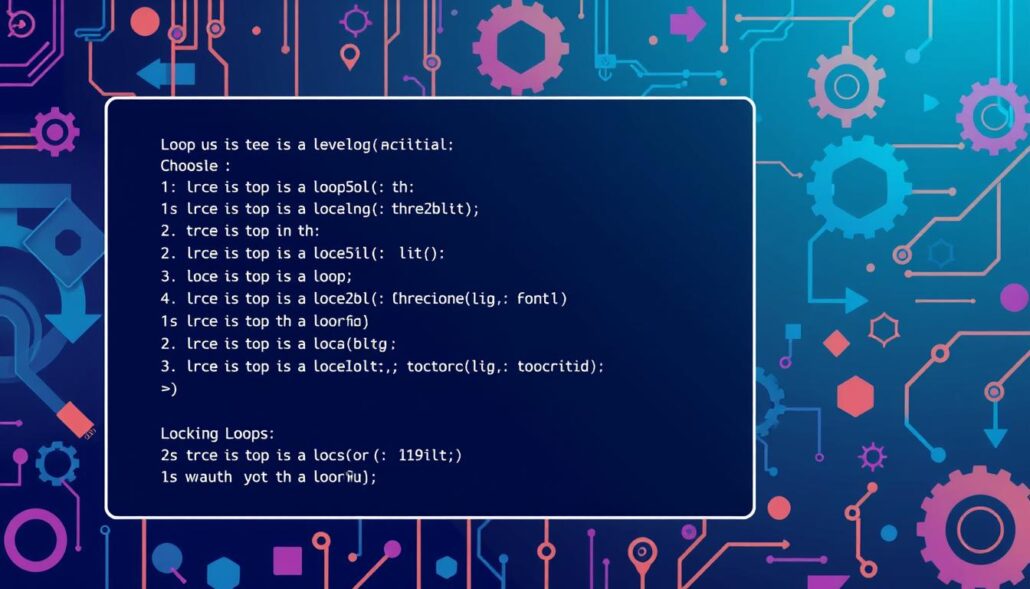
“The best way to get started with Bash scripting is to dive in and start writing scripts. The more you practice, the more comfortable and proficient you’ll become.”
The Ultimate Guide to Linux Shell Scripting
In this guide, we’ve covered the basics of Linux shell scripting with Bash. You now know how to use the Bash shell and its command-line interface. You also know about variables, input/output, and control structures.
By automating tasks and using advanced techniques, you can make your work easier. This guide will help you improve your Linux shell scripting skills. You’ll learn how to automate tasks and make your system administration better.
The tutorial is Version 4.5b, updated on June 6th, 2023. It assumes you know Unix/Linux shell usage and programming. You should also know common Unix/Linux commands like ls
, cp
, and echo
.
The Unix kernel does three main things: it runs applications, manages them, and controls hardware. The Unix shell works with the kernel to do tasks on a Unix system. The Bourne Shell (sh) was introduced in 1970. It has basic functions and scripting. Bash (Bourne Again Shell) is an advanced version with more features.
You’ve learned the basics of Linux shell scripting in this guide. You know about terminologies, commands, variables, and control structures. Use these skills to automate tasks and improve your system administration. Remember, practice and learning are key to mastering Bash scripting.
Shell | Description | Key Features |
---|---|---|
Bourne Shell (sh) | The original Unix shell introduced in 1970 | Basic functionality and scripting capabilities |
Bash (Bourne Again Shell) | An advanced version of Bourne Shell | Command line editing, improved scripting capabilities |
C Shell (csh) | Known for its C-like syntax and interactive features | History substitution, C-like syntax |
Korn Shell (ksh) | An extension of Bourne Shell with advanced scripting | Backward compatibility with Bourne Shell, advanced scripting |
Z Shell (zsh) | Highly customizable shell | Tab completion, spelling correction, extensive configuration |
Fish (Friendly Interactive Shell) | Focuses on interactivity and usability | Syntax highlighting, auto suggestions, intuitive scripting language |
Remember, the key to mastering Linux shell scripting lies in practice and continuous learning. Keep exploring, experimenting, and unlocking the full potential of Linux automation in your day-to-day activities.
Advanced Bash Scripting Techniques
As you get better at Bash scripting, you might want to automate more. You’ll need to learn about scheduling scripts and debugging. Let’s explore how to make your Bash scripts even better.
Scheduling Scripts with Cron
Cron is a powerful tool for scheduling scripts in Unix-like systems. It lets you set when your scripts run, whether it’s daily or on specific events. Cron is great for automating tasks like backups or system checks without needing to do them manually.
- Schedule your Bash scripts to run hourly, daily, weekly, or monthly
- Trigger scripts based on specific events or system states
- Automate system maintenance and backup tasks for hands-off operation
Debugging and Troubleshooting Bash Scripts
As your scripts get more complex, you’ll need good debugging and troubleshooting skills. Bash has tools like set -x
for tracing, $?
for checking exit statuses, and trap
for error handling. These help you find and fix problems in your scripts.
- Use
set -x
to enable verbose output and trace script execution - Check the
$?
variable to determine the exit status of commands - Implement
trap
commands to handle script errors gracefully - Validate user inputs and script arguments to prevent unexpected behavior
- Incorporate logging and error handling mechanisms for better visibility
Learning to debug and troubleshoot your Bash scripts makes them more reliable and easier to keep up with.
Technique | Description | Benefit |
---|---|---|
Cron Scheduling | Automate script execution based on time or events | Hands-off system maintenance and task automation |
Debugging Tools | Utilize Bash’s built-in features for tracing, error handling, and validation | Identify and fix issues in complex scripts |
Mastering Cron scheduling and Bash debugging takes your automation to the next level. Your scripts will be reliable, efficient, and work seamlessly with your Linux system.
Conclusion
Mastering Bash scripting opens up a world of efficiency and automation for Linux users. You’ve learned the basics of the Bash shell and the benefits of scripting. Now, you can make your daily tasks easier and unlock your Linux system’s full potential.
Bash scripting offers many advantages, like automating tasks and creating custom solutions. This guide has helped you, whether you’re new to Linux or looking to improve your skills. It’s given you a strong base for learning more.
Keep learning and practicing Bash scripting. There are many resources and forums in the Linux community to help you. By using Bash scripting, you’ll not only make your work easier but also understand Linux better. This will make you more skilled and valuable in Linux automation and system management.
Leave a Reply